Using the Shell
We've gone over a lot of definitions and technologies. It is time to dive into using them.
This article covers the shell more in-depth.
Linux and Terminal
Linux and Mac come with a program called "Terminal". The program allows you to type in commands to the local computer and remote computers.
Terminal
Find and open the "Terminal" application. It looks like this:

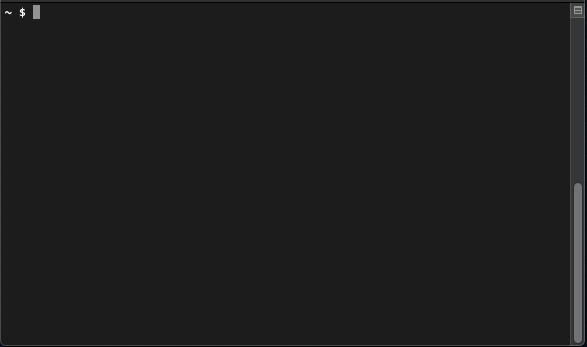
Is terminal a shell?
Terminal is running a specific shell that it is configured to run. To see which shell it is using, open terminal and run the command:
ps -p $$
On a mac configured to use Zsh I see terminal running zsh:
% ps -p $$
PID TTY TIME CMD
26000 ttys002 0:00.02 -zsh
And on HE's V3 platform configured to use bash:
$ ps -p $$
PID TTY TIME CMD
17700 pts/0 00:00:00 bash
Windows and PuTTY
If you ask 'how to use SSH on Windows' to anybody other than a Windows power user, they'll tell you Windows doesn't come with SSH. It requires a program called PuTTY.
PuTTY
Download PuTTY from https://www.chiark.greenend.org.uk/~sgtatham/putty/latest.html.
Find and open the download. It looks like this:
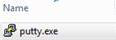
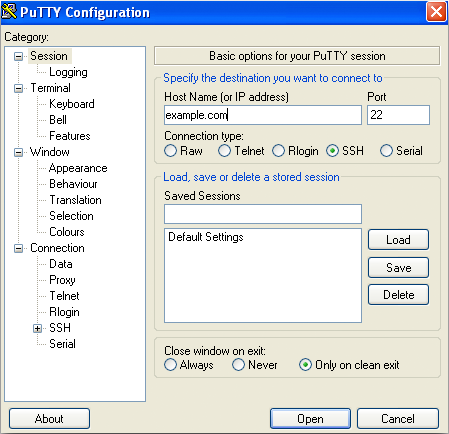
What shell does PuTTY use?
PuTTY establishes an SSH connection to a remote server. The remote server runs the shell associated with the user.
On HE's V3 platform configured to use bash:
$ ps -p $$
PID TTY TIME CMD
17700 pts/0 00:00:00 bash
Secure Shell (SSH)
We've talked about shells -- programs that run locally on computers that humans can use to give the computer commands -- and we've also talked about SSH. But what is SSH? SSH stands for Secure Shell. SSH is used to establish an encrypted connection to a remote shell.
With SSH, we can be on our home computer and issue commands to the remote server running our website.
For all the telnet users out there thinking, "that's just telnet," you're right. But telnet sends everything, including usernames and passwords over the internet in plaintext for all to read. Anyone still using telnet should switch to SSH for the security it provides.
Logging In
SSH connections can be authenticated using a username/password combination or an SSH key.
SSH keys are assymetric public/private key pairs that can log a user into an SSH session without typing in the password. They are good for users that don't want to type in passwords. They are great for tasks where using a password is undesirable, like in a script.
Generating a key
Cryptography is complex and generating SSH keys looks daunting, but we don't need to know cryptography theory, just how to use it as a tool. Anybody using SSH should familiarize themselves with creating and using SSH keys.
Which side is which?
Public/private key pairs can be generated anywhere and moved anywhere, but private keys are named "private" keys because they are supposed to be kept private. The best way to keep them private is to generate them on the computer they are to reside on and then never touch them again. So, the first step to generating a key is to log in to the computer that it will identify.
In this example, I am creating a key that will identify my local computer. The private key will reside on my local computer. I will never move the private key to another computer. I won't email the private key. I won't post the private key anywhere. I won't copy the private key. I will not share the private key with anyone or leave it anywhere that it can be seen by others. THE PRIVATE KEY IS PRIVATE.
I will share the public key with a remote computer that adds it to a list of authorized keys. I can share the public key because it is meant to be public. It is not secret. It can be shared with anyone. It can be viewed. It can be stolen. Announce it to the world. THE PUBLIC KEY IS PUBLIC.
Then, when I attempt to log in from my local computer to the remote computer, the remote computer sees my (public) key is authorized and challenges the local computer to answer a question that is nearly impossible to answer without knowing the private key. Since the local computer knows the private key, it answers correctly and is allowed access to the remote machine.
ssh-keygen command
On the computer this key will identify, my local computer in this
example, use the ssh-keygen
command to generate a
public/private key pair:
% ssh-keygen -t ed25519
Generating public/private ed25519 key pair.
Enter file in which to save the key (/home/example/.ssh/id_ed25519): /home/example/.ssh/id_net_example
Enter passphrase (empty for no passphrase):
Enter same passphrase again:
Your identification has been saved in id_net_example
Your public key has been saved in id_net_example.pub
ssh-keygen
tells the computer to generate an ssh key pair.
-t ed25519
says to generate an "ed25519" key; the
currently recommended key type.
The computer responds that it is generating a key pair and prompts
for a name to give the key. Name the key after what it will be used
for and make sure to save it in the ~/.ssh
folder.
This key will be used to log into the remote host, example.net, so
I saved it to ~/.ssh/id_net_example
.
The computer will ask if the key should have a password. We are setting up keys for passwordless authentication so leave the passphrase blank.
Now you should have two files. id_net_example
is the
private key. DO NOT SHARE THE PRIVATE KEY.
id_net_example.pub
is the public key. The public key
is for sharing.
ssh-copy-id
The public key needs to be added to a remote server's list of
authorized keys. ssh-copy-id
does that.
% ssh-copy-id -i ~/.ssh/id_net_example.pub example@example.net
/usr/bin/ssh-copy-id: INFO: Source of key(s) to be installed: "/home/example/.ssh/id_net_example.pub"
/usr/bin/ssh-copy-id: INFO: attempting to log in with the new key(s), to filter out any that are already installed
/usr/bin/ssh-copy-id: INFO: 1 key(s) remain to be installed -- if you are prompted now it is to install the new keys
example@example.net's password:
Number of key(s) added: 1
Now try logging into the machine, with: "ssh 'example@example.net'"
and check to make sure that only the key(s) you wanted were added.
The -i ~/.ssh/id_net_example.pub
specifies the key to
authorize.
example@example.net
is the remote user and host to copy
the public key to. It is the host that our local computer will be allowed
to log in to.
I am prompted for the password to the remote machine so that it can log in and append the public key to the file containing authorized keys.
If I logged in successfully, it will suggest I try logging in with the key. It also suggests checking that the correct key was added. Let's do that now.
% ssh -i ~/.ssh/id_net_example example@example.net
No mail
$
$ tail -n1 ~/.ssh/authorized_keys
ssh-ed25519 AAAAC3NzaC1lZDI1NTE5AAAAIOa0kGKz44aneDlN2m71kbSQOPkzr0CPu7jQbxT0YlIl local-user@my.local
I told SSH to specifically use the key I generated earlier. I can leave that out and it will try each key until one works.
The key worked. I was logged in without being prompted for a password.
The ssh-copy-id
command appends the public key to the ~/.ssh/authorized_keys
file on the remote server. I checked the last line of the file and there's the key I generated. So ssh-copy-id
copied the correct key to the remote server and now I can log in without entering my password.
Shell Types
There are many different shells available to use. While they can be very similar, it is important to recognize that all shells are not the same and commands may work differently across different shells.
Bash
There are many different shells and they vary from shell to shell. The HE platform's default shell is the Bourne Again Shell (bash). When logging into the server with SSH the server provides an interactive bash shell (unless you were to change it).
Zsh
Zsh is another popular shell. It has tons of features. It is built with users in mind. Apple recently switched the default shell on its OS from Bash to Zsh.
Shell Basics
Start with the basics and the rest will come later.
Controls
Shell controls take some practice. The user interacts with the shell with the keyboard by entering commands and pressing keyboard combinations.
History
Up and down goes through the commands that have been run. Rather than retyping a command, use up to quickly access it again.
Autocomplete
Tab is your best friend in the shell. It will try to autocomplete most anything. Press tab twice to see possible options. Learn to use tab excessively.
Cursor Position
Left and right will move the cursor position. Jump to the front of a line with ctl + a and jump to the end of a line with ctl + e.
Commands
Type a command into the terminal window and press enter to execute that command.
Manuals and Information
There are varying degrees of help available while using shell. They are not always available, but it is important to know the places to check.
Man
man {command}
shows the manual for {command}.
Info
info {command}
when available shows a more detailed edition of the manual.
Help
{command} --help
usually gives a very brief overview of using a command. If more explanation is necessary, use man
or info
.